
“Unravel the unexpected—how far will you go when the destination is a mystery?”
Technical Breakdown
The Problem
Create a system for an NPC to move to a new position on a grid. It should find the shortest path around obstacles or return a negative value if no path exists.
The Solution

Check Neighbours
The pathfinder checks the four nearby tiles and calculates three values for each:
H: estimated distance to the target
G: distance from the start
F: total cost (H + G)
It then moves to the tile with the lowest F cost and repeats the process.
Iterate through neighbours
Repeat neighbour check process until finding destination
Record connecting nodes
Each node stores a link to the node that created it, allowing each new node to trace back to its origin.
Calculate returning path
Starting at the target node, trace back through each connecting node to the start. Convert this path into an array of coordinates in reverse order to go from start to target.
Move through coordinates
Input the array of coordinates into NPC movement.
// Finds a path from the start position to the target position
public static List<Vector2Int> FindPath(Vector2Int startPosition, Vector2Int targetPosition)
{
// Validates the target position before proceeding to find the path
if (!IsTargetValid(targetPosition)) return null;
// Initializes start and target nodes
PathNode startNode = new PathNode(startPosition);
PathNode targetNode = new PathNode(targetPosition);
// Performs path search and returns the result
PathNode finalNode = SearchPath(startNode, targetNode);
return finalNode != null ? BuildPath(finalNode) : null;
}
// Searches for a path using the A* algorithm
private static PathNode SearchPath(PathNode startNode, PathNode targetNode)
{
// Initializes lists for open and closed nodes and a dictionary for node references
var openList = new List<PathNode> { startNode }; // Nodes to be evaluated
var closedList = new HashSet<PathNode>(); // Nodes already evaluated
var vecNodeDict = new Dictionary<Vector2Int, PathNode>
{
[startNode.Position] = startNode // Store start node
};
int searchCount = 0; // Limit search iterations
while (searchCount < 1000 && openList.Count > 0)
{
// Gets node with the lowest F cost
PathNode current = GetLowestFCostNode(openList);
// Checks if the target has been reached
if (IsTargetNode(current, targetNode))
return current;
openList.Remove(current); // Moves current node to closed list
closedList.Add(current);
// Evaluates neighbors of the current node
foreach (var neighbor in GetNeighbourNodes(current))
{
if (closedList.Contains(neighbor)) continue; // Skips evaluated neighbors
// Updates costs of the neighbor
UpdateNodeCosts(current, neighbor, targetNode);
// Adds to open list if not already present
if (!openList.Contains(neighbor))
{
openList.Add(neighbor);
vecNodeDict[neighbor.Position] = neighbor; // Stores neighbor in dictionary
}
}
searchCount++; // Increments search count
}
return null; // Returns null if no path found
}
// Updates the G and H costs for a neighbor node
private static void UpdateNodeCosts(PathNode current, PathNode neighbor, PathNode targetNode)
{
float gCost = current.G + 1; // Cost from start to current node
float hCost = GetDistance(neighbor, targetNode); // Heuristic cost to target
// Updates costs if this path to neighbor is better than any previous one
if (gCost < neighbor.G)
{
neighbor.SetConnection(current); // Sets current as connection
neighbor.SetCosts(gCost, hCost); // Updates costs
}
}
// Builds the path from the final node to the start node
private static List<Vector2Int> BuildPath(PathNode finalNode)
{
var pathCoords = new List<Vector2Int>();
// Traverses back through the connections to build the path
while (finalNode != null)
{
pathCoords.Add(finalNode.Position);
finalNode = finalNode.Connection; // Moves to the next node in the path
}
pathCoords.Reverse(); // Reverses to get the path from start to end
return pathCoords;
}
// Gets the node with the lowest F cost from the open list
private static PathNode GetLowestFCostNode(List<PathNode> openList) =>
openList.OrderBy(node => node.F).First();
// Checks if the current node is the target node
private static bool IsTargetNode(PathNode current, PathNode targetNode) =>
current.Position == targetNode.Position;
// Retrieves the neighboring nodes of the current node
private static List<PathNode> GetNeighbourNodes(PathNode node)
{
var neighbors = new List<PathNode>();
var directions = new Vector2Int[] {
new Vector2Int(-1, 0), new Vector2Int(1, 0), new Vector2Int(0, 1), new Vector2Int(0, -1)
};
// Checks each direction for valid neighbor nodes
foreach (var dir in directions)
{
Vector2Int newPos = node.Position + dir;
if (TileOccupied(newPos)) continue; // Skips occupied tiles
// Creates a new neighbor node
var neighborNode = new PathNode(newPos);
neighborNode.SetConnection(node); // Sets current node as connection
neighbors.Add(neighborNode); // Adds to neighbors list
}
return neighbors;
}
// Checks if the specified tile is occupied
private static bool TileOccupied(Vector2Int position) =>
Physics2D.OverlapBox(position, Vector2.one * 0.2f, 0f) != null;
// Calculates the Manhattan distance between two nodes
private static float GetDistance(PathNode a, PathNode b) =>
Mathf.Abs(a.Position.x - b.Position.x) + Mathf.Abs(a.Position.y - b.Position.y);
// Validates if the target position is unoccupied
private static bool IsTargetValid(Vector2Int targetPos) =>
Physics2D.OverlapBox(targetPos, Vector2.one * 0.4f, 0f) == null;
The Problem

Technical Artists need an in-engine tool to quickly create and iterate tileset maps while dynamically controlling interaction settings of tile types
The Solution
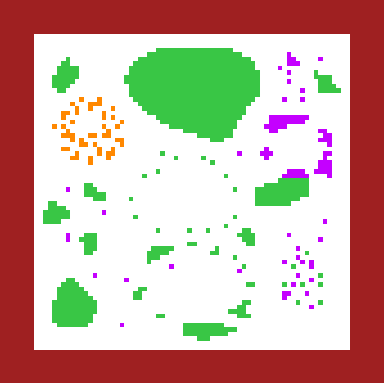
Generate Map Texture
Draw a simple pixel map in any program

Assign Tiles to Colours
Create a Map Generator Asset and assign tiles to colours on the map using the colour picker tool

Configure Tiles
In Tile Prefab, the settings of the tile can be configured, such as the types of interactions, if its traverseable, etc

Scene Map Generator
Add a SceneMapGenerator Prefab to a scene. Assign the parent transform to hold tiles and the Map Generator Asset to use.
Generate Map
Scene Map Generator will read the pixel colours of the map texture, find any Tiles assigned to that colour, and spawn it in the world position coordinate equivalent to the pixel.
The Problem
Player needs to move through world.
This movement should not 'translate' but 'snap' from grid point to grid point.
Player should stop before entering a tile pre-occupied by another tile, unless that tile is traverseable.
The Solution
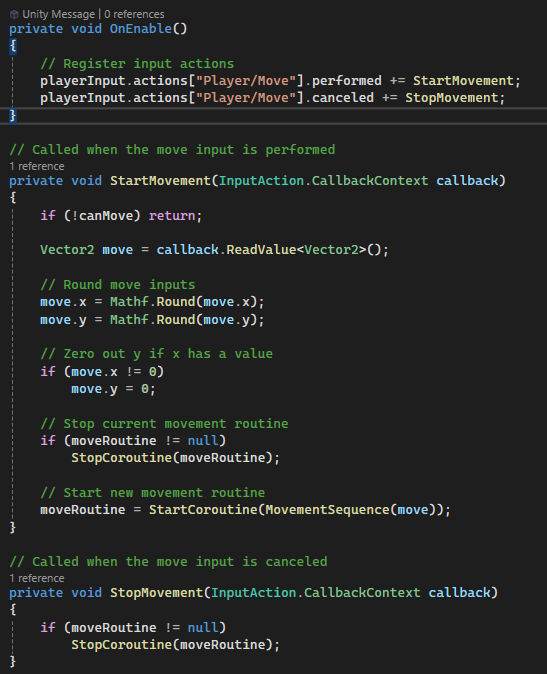
Input Handling
Player input triggers movement actions. Each time movement is performed or canceled, an event fires to update related components like animations.
Coroutine movement means logic is only called when its needed instead of every frame.

Collision Checking
Before each move, a check ensures the next tile is either empty or marked as “traversable.”
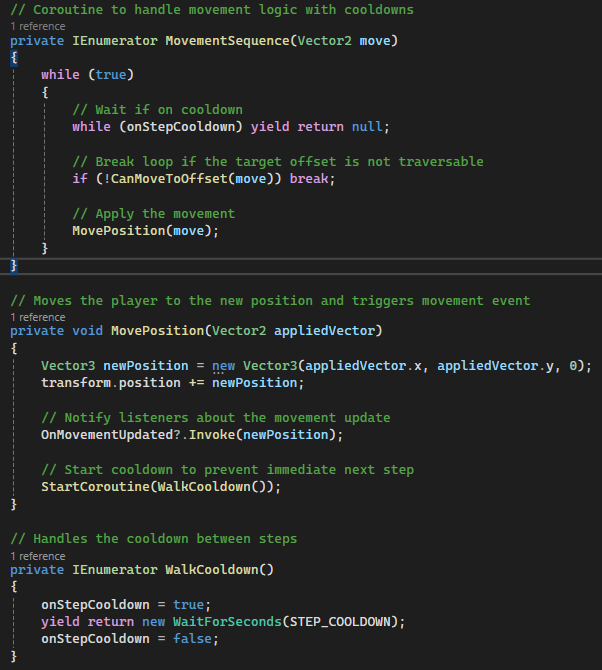
Sequential Movement
Movement is handled in a sequence, snapping the player to the next grid point only after cooldown. This allows for smooth, step-by-step progression on the grid.
public Action<Vector2> OnMovementUpdated; // Event to notify listeners about movement updates
private bool canMove = true;
private bool onStepCooldown = false;
private PlayerInput playerInput;
private Coroutine moveRoutine; // Coroutine for managing continuous movement
private const float STEP_COOLDOWN = 0.2f;
private void Awake()
{
playerInput = GetComponent<PlayerInput>();
}
private void OnEnable()
{
// Register input actions
playerInput.actions["Player/Move"].performed += StartMovement;
playerInput.actions["Player/Move"].canceled += StopMovement;
}
private void OnDisable()
{
// Unregister input actions
playerInput.actions["Player/Move"].performed -= StartMovement;
playerInput.actions["Player/Move"].canceled -= StopMovement;
}
// Called when the move input is performed
private void StartMovement(InputAction.CallbackContext callback)
{
if (!canMove) return;
Vector2 move = callback.ReadValue<Vector2>();
// Round move inputs
move.x = Mathf.Round(move.x);
move.y = Mathf.Round(move.y);
// Zero out y if x has a value
if (move.x != 0)
move.y = 0;
// Stop current movement routine
if (moveRoutine != null)
StopCoroutine(moveRoutine);
// Start new movement routine
moveRoutine = StartCoroutine(MovementSequence(move));
}
// Called when the move input is canceled
private void StopMovement(InputAction.CallbackContext callback)
{
if (moveRoutine != null)
StopCoroutine(moveRoutine);
}
// Coroutine to handle movement logic with cooldowns
private IEnumerator MovementSequence(Vector2 move)
{
while (true)
{
// Wait if on cooldown
while (onStepCooldown) yield return null;
// Break loop if the target offset is not traversable
if (!CanMoveToOffset(move)) break;
// Apply the movement
MovePosition(move);
}
}
// Checks if the position offset is traversable
private bool CanMoveToOffset(Vector2 targetOffset)
{
Vector2 targetPosition = (Vector2)transform.position + targetOffset;
Collider2D col = Physics2D.OverlapBox(targetPosition, new Vector2(0.2f, 0.2f), 0);
if (!col) return true; // Return true if no collision detected
// Check if the tile is traversable
Tile tile = col.GetComponent<Tile>();
return tile != null && tile.isTraverseable;
}
// Moves the player to the new position and triggers movement event
private void MovePosition(Vector2 appliedVector)
{
Vector3 newPosition = new Vector3(appliedVector.x, appliedVector.y, 0);
transform.position += newPosition;
// Notify listeners about the movement update
OnMovementUpdated?.Invoke(newPosition);
// Start cooldown to prevent immediate next step
StartCoroutine(WalkCooldown());
}
// Handles the cooldown between steps
private IEnumerator WalkCooldown()
{
onStepCooldown = true;
yield return new WaitForSeconds(STEP_COOLDOWN);
onStepCooldown = false;
}
// Disables movement, stopping the movement coroutine if active
public void StopMovement()
{
canMove = false;
if (moveRoutine != null)
StopCoroutine(moveRoutine);
}
// Enables movement
public void StartMovement()
{
canMove = true;
}
The Problem
Player needs to be able to interact with certain tiles.
Conditions:
(3 Trees should show 1 cut tree button)
The Solution
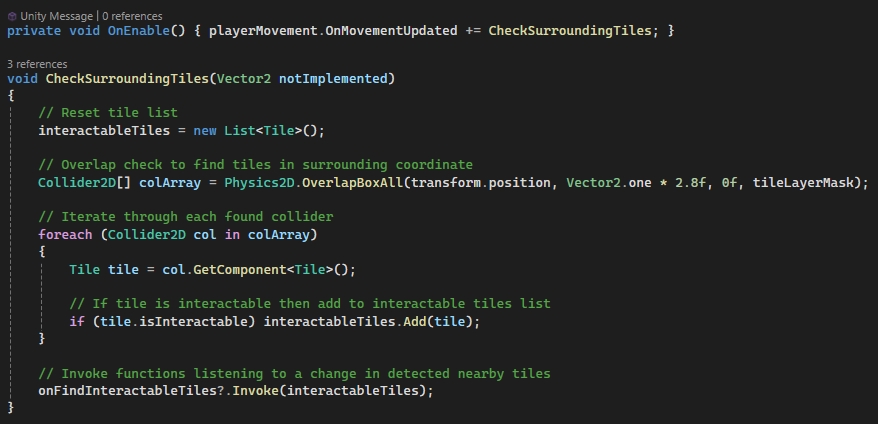
Detect Tiles
Check the surrounding coordinates for interactable tiles
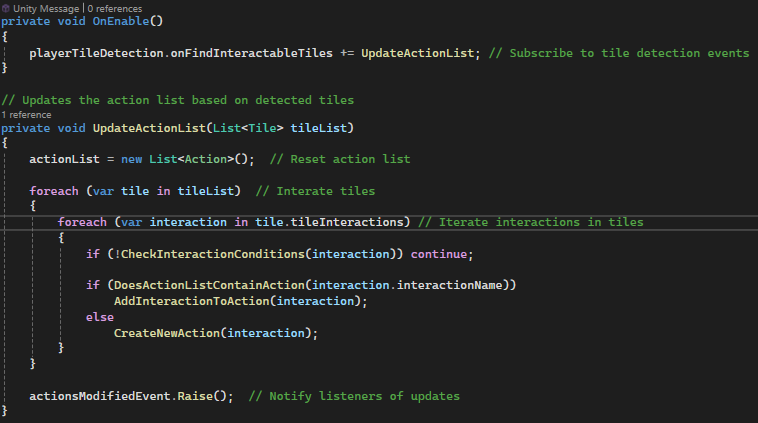
Configure Actions
Group the same interactions under one 'action'.
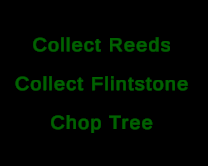
Display Buttons
Update the onscreen buttons with possible actions and bind action results to press.
[RequireComponent(typeof(PlayerMovement2D))]
public class PlayerTileDetection : MonoBehaviour
{
PlayerMovement2D playerMovement;
[SerializeField] LayerMask tileLayerMask;
[SerializeField] List<Tile> interactableTiles;
public GameEvent actionsModified;
public event Action<List<Tile>> onFindInteractableTiles;
private void Awake()
{
playerMovement = GetComponent<PlayerMovement2D>();
}
public void Start()
{
RecheckCurrentPosition();
}
private void OnEnable()
{
playerMovement.OnMovementUpdated += CheckSurroundingTiles; // Subscribe tile check
}
private void OnDisable()
{
playerMovement.OnMovementUpdated -= CheckSurroundingTiles; // Unsubscribe tile check
}
void CheckSurroundingTiles(Vector2 notImplemented)
{
// Reset tile list
interactableTiles = new List<Tile>();
// Overlap check to find tiles in surrounding coordinate
Collider2D[] colArray = Physics2D.OverlapBoxAll(transform.position, Vector2.one * 2.8f, 0f, tileLayerMask);
// Iterate through each found collider
foreach (Collider2D col in colArray)
{
Tile tile = col.GetComponent<Tile>();
// If tile is interactable then add to interactable tiles list
if (tile.isInteractable) interactableTiles.Add(tile);
}
// Invoke functions listening to a change in detected nearby tiles
onFindInteractableTiles?.Invoke(interactableTiles);
}
public void RecheckCurrentPosition()
{
CheckSurroundingTiles(transform.position);
}
}
public class ConfigureNearbyTileActions : MonoBehaviour
{
[SerializeField] private PlayerTileDetection playerTileDetection; // Detects nearby interactable tiles
public List<Action> actionList; // Actions derived from tile interactions
public GameEvent actionsModifiedEvent; // Event raised when action list updates
private void OnEnable()
{
playerTileDetection.onFindInteractableTiles += UpdateActionList; // Subscribe to tile detection events
}
private void OnDisable()
{
playerTileDetection.onFindInteractableTiles -= UpdateActionList; // Unsubscribe to tile detection events
}
// Updates the action list based on detected tiles
private void UpdateActionList(List<Tile> tileList)
{
actionList = new List<Action>(); // Reset action list
foreach (var tile in tileList) // Interate tiles
{
foreach (var interaction in tile.tileInteractions) // Iterate interactions in tiles
{
if (!CheckInteractionConditions(interaction)) continue;
if (DoesActionListContainAction(interaction.interactionName))
AddInteractionToAction(interaction);
else
CreateNewAction(interaction);
}
}
actionsModifiedEvent.Raise(); // Notify listeners of updates
}
// Checks if all interaction conditions are met
private bool CheckInteractionConditions(Interaction interaction)
{
foreach (var condition in interaction.interactionConditions)
{
if (!condition.CheckCondition()) return false;
}
return true;
}
// Returns true if action with the specified name exists
private bool DoesActionListContainAction(string actionName)
{
return actionList.Exists(action => action.actionName == actionName);
}
// Creates a new action with the given interaction
private void CreateNewAction(Interaction interaction)
{
var newAction = new Action
{
actionName = interaction.interactionName,
actionInteractions = new List<Interaction> { interaction }
};
actionList.Add(newAction);
}
// Adds interaction to an existing action
private void AddInteractionToAction(Interaction interaction)
{
foreach (var action in actionList)
{
if (action.actionName == interaction.interactionName)
{
action.actionInteractions.Add(interaction);
return;
}
}
}
}